A simple Node.js REST server simulator for Testing
Problem
You are developing a client application (AngularJS) and need to retrieve data from a REST API service. However, you often lack access to the remote service, or it doesn’t have the required data for your tests (e.g., dynamically generated data).
A direct request to a test .json
file is not possible due to browser security restrictions—browsers cannot directly access the file system.
Solution – Concept
A client application can send a GET request to a local server, which then serves a JSON file as a response.
This local server can be implemented in Node.js, which handles HTTP requests and serves static files.
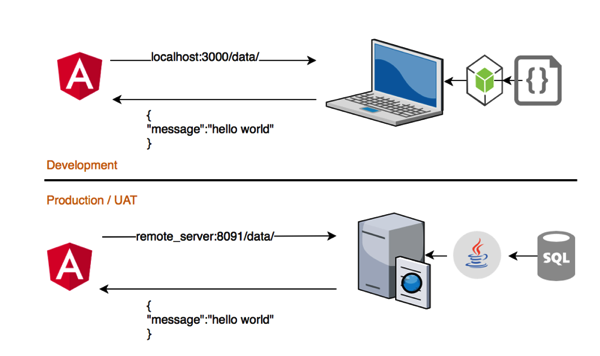
Solution – Implementation
The code and installation guide are available here:
🔗 GitHub Repository
Project Structure
The project follows a simple structure:
Example Response
Here’s an example of a JSON response:
Solution – Details
The server.js
file creates an HTTP server that listens for requests.
It uses a loaderModule
, which contains a class responsible for retrieving the JSON data.
server.js
// Import the function from the module
var loaderModule = require('./ResponseLoader.js');
// Create an instance of the prototype
var loader = new loaderModule("loader");
function requestService(request, response) {
// URL is the filename without extension
var url = request.url;
// Handle home page request
if (url == "/") {
url = "/index";
}
// Load the static JSON file
loader.load(url);
// Prepare the HTTP response
response.statusCode = 200;
response.setHeader('Content-Type', 'application/json');
// Send JSON content
response.end(loader.getJson());
}