Migration from Kotlin to Java, notes
Many projects migrate Java to Kotlin, some go from Kotlin to Java.
These are some notes about my experience in the migration of a Kotlin project to Java. These are technical notes, not an evaluation of the two languages.
đ ď¸ This post is still in progress. I'm updating it during the migration process.
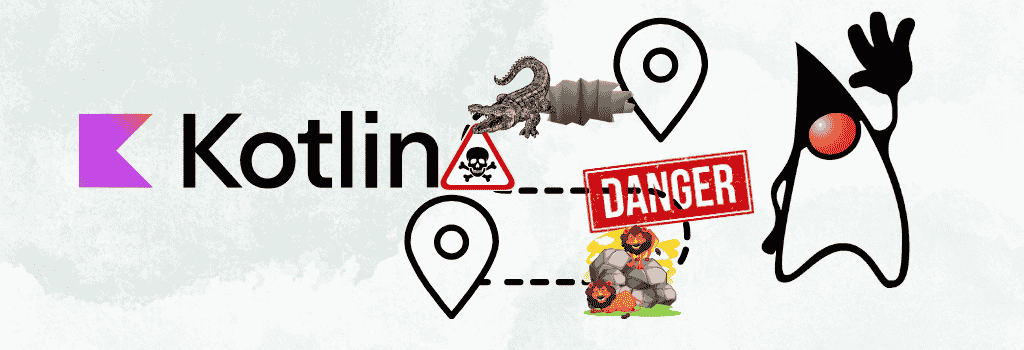
Cool Features That I'd Like in Java
There are many differences between the two languages. What I miss the most while developing in Java:
-
Nominal parameters
Many languages have this feature â in Java we donât and wonât have it. When working with big data objects, itâs easy to write constructor parameters in the wrong order. -
Copy
In Kotlin, objects can be copied while modifying some properties:
đ Kotlin Data Class Copying
Null Safety
This was a big topic during discussions, but in reality, we didnât have many problems.
Sure, Java doesnât come with built-in null safety, but often the issue is exaggerated. Just because a method accepts null
doesnât mean the application logic will pass a null value.
To reassure the team, we allowed the use of Lombok's @NotNull
annotation â this helped clarify which parameters could not be null.
For method return types, we preferred to use Optional
when nulls were allowed.
With Kotlin interop, using Optional
brought some small complexities when integrating with existing functions.
Annoying Small Issues
foreach
- In Java,
return
continues to the next item in the loop. - In Kotlin,
return
exits the loop â the equivalent isreturn@foreach
.
typealias
- In Kotlin, you can define an alias for a type.
- In Java, this doesnât exist. We just used the original type.
- Alternatively, we could create new classes, but that wasn't common in the original project.
Variable â Type Inference
- We preferred explicit types in Java.
- When Kotlin code overused type inference, we used
var
in Java. - Our team wanted to see the types rather than guess what kind of object was being used.
Lombok Issues
During the transition, we faced issues with Lombok:
- Kotlin struggled to interpret Lombok-generated getters as properties.
- As a result, we had to manually add getters in our Java
@Data
classes. - IntelliJ constantly complained otherwise.
Inline Functions
In Kotlin, small methods were often inlined. For example:
fun findExternalProducts() =
productService.findProducts().filter { it.type in ProductType.external }
Migrating these wasnât too hard â but type inference was the pain point.
When type information is stripped out, the developer has to "think and search" for what kind of data is being manipulated. Easy in simple cases, harder in complex chains.
Kotlin and Jackson
We had to migrate a lot of infrastructure code, including JSON-based components.
Kotlin and Jackson donât play well together by default:
- Kotlin data classes donât have no-arg constructors unless you define default values.
- Jackson doesnât know how to map constructor parameters without that.
- With complex objects, this wasnât workable.
A workaround:
Add another plugin to make them play nice:
final ObjectMapper objectMapper = new ObjectMapper()
.registerModule(new KotlinModule.Builder().build());
With Gradle:
implementation("com.fasterxml.jackson.module:jackson-module-kotlin")
Collections
Kotlin has many convenient shorthand methods for collections:
Example: Intersection
collectionOne.intersect(collectionTwo)
Java equivalent:
collectionOne.stream()
.filter(collectionTwo::contains)
.collect(Collectors.toSet());
Not too complex, but during migration, you have to stop and think how to "translate" it.
Example: Adding two immutable collections
In Kotlin:
collectionOne + collectionTwo
In Java:
List<Data> allData = new ArrayList<>(collectionOne);
allData.addAll(collectionTwo);
return allData;
Hibernate
There are a lot of online discussions criticizing Hibernate in Kotlin.
We didnât face many issues. Despite their different philosophies (immutability vs. mutability), they can work well together â especially with the help of 2â3 helper plugins.
Migrating from Kotlin to Java? Hibernate had fewer frictions.
IDE Lock-in
This wasnât a problem for us, but migrating to Java allowed the frontend team to work more directly with backend code.
Our frontend team used Visual Studio Code (donât ask me why đ
).
Kotlin has no official support for VS Code, nor plans for it. Itâs pretty much a JetBrains-only language.
Unsurprisingly, IntelliJ is very eager to migrate Java â Kotlin.
But the opposite (Kotlin â Java) is not supported.
Some small migration steps
Remove typealias - Easy
In Kotlin we can have typealias
like:
typealias Name = String
or
typealias HidingComplexity = Map<Name, Map<Card, Shop>>
After this you can use the type Name
to replace String. This, on one side, can help the readability of the code, on the other side, in case of excessive utilisation can create confusion because the types used are not immediately identifiable.
Java doesn't support directly this feature. For the migration to Java we have to remove these aliases and replace them with the original type.
Lombok issue - create getter and setters
We use Lombok in our project, this helps to reduce the ceremonies of the out-of-the-box Java.
With mixed code Kotlin - Java, Kotlin doesn't recognize the Lombok annotations. Probably is possible to have some build configurations that allow a smooth integration of Lombok with Kotlin.
If you have private fields of classes annotated with @Getter
or @Data
, in Java you can use the standard get
method to access the value.
Kotlin automatically remove the get
from the method and it tries to access directly the field, doing this it will fail during the compilation because your filed is private
and it cannot be accessed.
Kotlin updated to the last version
To simplify the migration it's better to use the latest version of kotlin. Kotlin is moving quickly and maybe some support to feature that you need during the migration can be present in the latest version.
Kotlin will tell you if you are trying to use features that are more recent: e.g. The feature "references to synthetic java properties" is only available since language version 2.1
(we got this when we migrated getter and setters using version 1.9).
enum
This is another easy step, migrate enum class
of Kotlin to Java. Pretty straightforward e.g.:
enum class DevSkills(val skill: String) {
KOTLIN("kotlin");
}
to
import lombok.RequiredArgsConstructor;
@Getter
@RequiredArgsConstructor
public enum DevSkills {
KOTLIN("kotlin");
private final String skill;
}