@PostConstruct and @PreDestroy examples in Java
In Java, @PostConstruct
and @PreDestroy
are two annotations that are used to perform actions before and after an object's lifecycle.
These annotations are part of the JakartaEE standards (ex-Java EE) and are commonly used in Spring-based applications.
@PostConstruct
is used to annotate a method that needs to be executed after the object is constructed, but before it is put into service.
The method annotated with @PostConstruct
is guaranteed to be called only once during the lifetime of the object, and it is typically used to perform any initialization tasks that are required before the object can be used.
Here is an example:
public class MyService {
private SomeDependency someDependency;
private AnotherDependency anotherDependency;
@PostConstruct
public void init() {
// perform initialization tasks here
someDependency.init();
anotherDependency.init();
}
// other methods and dependencies
@PreDestroy
public void cleanup() {
// perform cleanup tasks here
someDependency.cleanup();
anotherDependency.cleanup();
}
}
In this example, the init() method is annotated with @PostConstruct
and it is called after the MyService
object is constructed.
In the init()
method, we perform any initialization tasks that are required before the object can be used.
Here, we call the init()
method on two dependencies that are required by MyService
.
@PreDestroy
is used to annotate a method that needs to be executed before the object is destroyed.
The method annotated with @PreDestroy
is guaranteed to be called only once during the lifetime of the object, and it is typically used to perform any cleanup tasks that are required before the object is destroyed.
Here is an example:
public class MyService {
private SomeDependency someDependency;
private AnotherDependency anotherDependency;
// other methods and dependencies
@PreDestroy
public void cleanup() {
// perform cleanup tasks here
someDependency.cleanup();
anotherDependency.cleanup();
}
}
In this example, the cleanup()
method is annotated with @PreDestroy
and it is called before the MyService
object is destroyed.
In the cleanup()
method, we perform any cleanup tasks that are required before the object is destroyed.
Here, we call the cleanup()
method on two dependencies that are required by MyService
.
Dependencies in Spring 3.x and Java 17: package javax.annotation does not exist
If you upgrade your application dependencies and you are still using javax.annotation.PostConstruct
you will get a nice error like:
package javax.annotation does not exist
it's because the javax
package has been removed in Java 11. Some websites suggest to import in your project:
<dependency>
<groupId>javax.annotation</groupId>
<artifactId>javax.annotation-api</artifactId>
<version>1.3.2</version>
</dependency>
... but like this you reintroduce the library (last version 2018) that Java decided to remove, better to use the new version of the library:
A much better solution is to use the updated versions of the annotation in your project:
import jakarta.annotation.PostConstruct;
import jakarta.annotation.PreDestroy;
probably you won't need extra dependencies, e.g. Spring Boot includes this dependency:
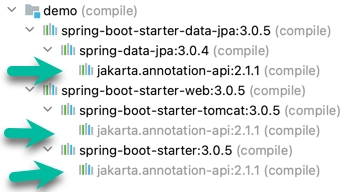
If you are using spring-boot-starter
this dependency comes without any effort on your side.